本文主要介绍spring事务(JDBC单数据库事务管理)的核心源码实现
1、核心类介绍
我们可以先看一下核心类图。
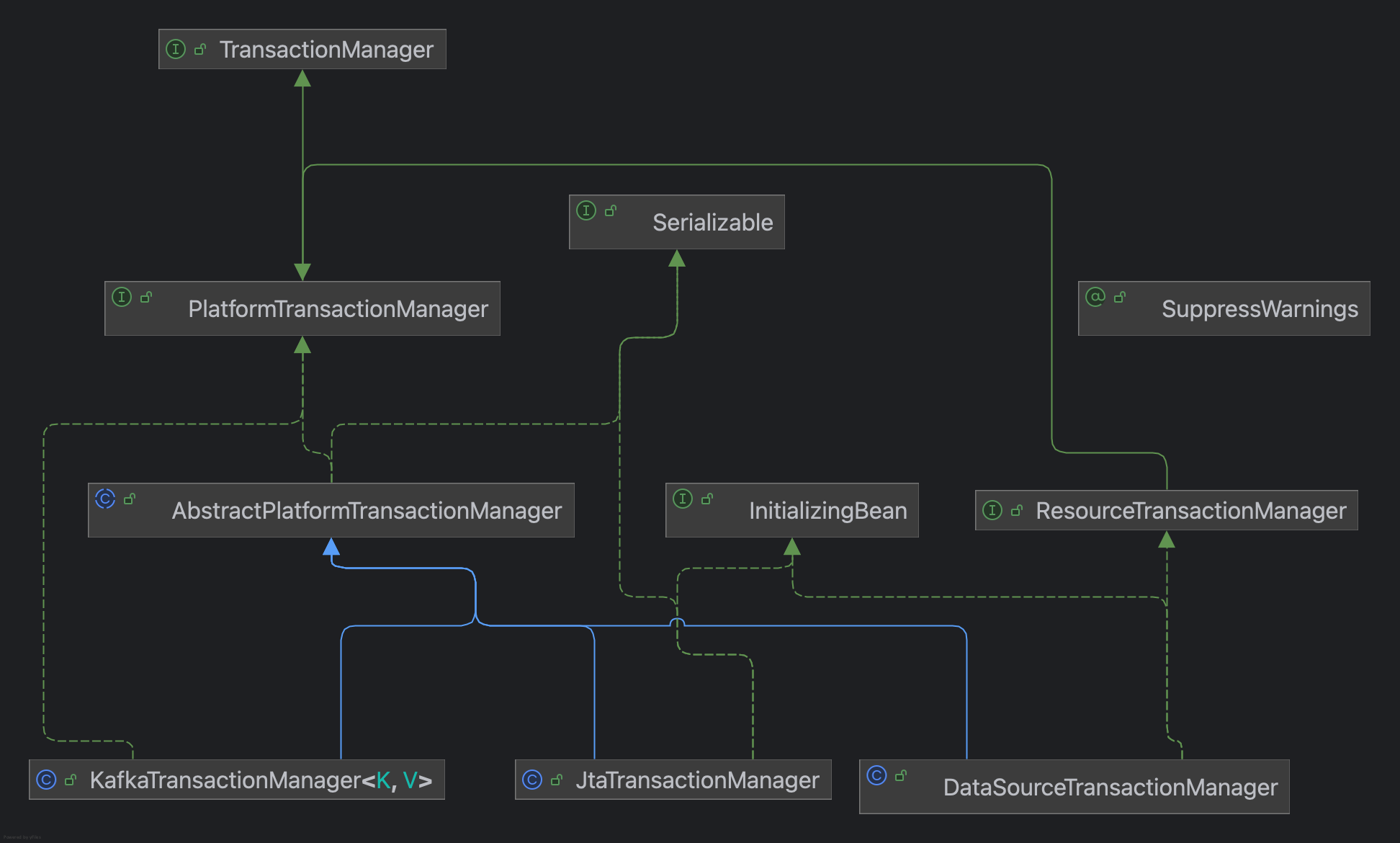
- PlatformTransactionManager
底层接口,定义事务方法1
2
3
4
5public interface PlatformTransactionManager extends TransactionManager {
TransactionStatus getTransaction( TransactionDefinition definition)
void commit(TransactionStatus status) throws TransactionException;
void rollback(TransactionStatus status) throws TransactionException;
} - AbstractPlatformTransactionManager
抽象类,实现PlatformTransactionManager接口,并定义了一些抽象方法比如doCommit、doRollback留给子类实现(这里使用了设计模式中的模板模式)。 - DataSourceTransactionmanager
JDBC单数据库事务管理器,基于Connection实现 - JtaTransactionManager
分布式事务管理器(本文不展开介绍) - KafkaTransactionManager
Kafka事务管理器(本文不展开介绍)
2、核心方法实现
2.1、getTransaction获取事务
- AbstractPlatformTransactionManager.getTransaction实现
1 |
|
- AbstractPlatformTransactionManager.handleExistingTransaction实现
该方法用于处理当前已存在事务的情况
1 | private TransactionStatus handleExistingTransaction( |
- AbstractPlatformTransactionManager.suspend
事务挂起
1 | protected final SuspendedResourcesHolder suspend( Object transaction)throws TransactionException { |
其中doSuspend会执行当前事务的挂起操作,AbstractPlatformTransactionManager抽象类doSuspend()会直接抛出异常,依赖于具体事务执行器的实现,DataSourceTransactionManager实现如下:
1 | protected Object doSuspend(Object transaction) { |
其中核心操作包含两步
1、当前事务清空ConnectionHolder
2、当前线程解绑datasource(ThreadLocal中移除resources对应的key)
TransactionSynchronizationManager
事务同步管理器,该类维护了多个线程本地变量ThreadLocal,如下所示:1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27public abstract class TransactionSynchronizationManager {
//事务资源
private static final ThreadLocal<Map<Object, Object>> resources =
new NamedThreadLocal<>("Transactional resources");
//事务同步信息
private static final ThreadLocal<Set<TransactionSynchronization>> synchronizations =
new NamedThreadLocal<>("Transaction synchronizations");
//当前事务名称
private static final ThreadLocal<String> currentTransactionName =
new NamedThreadLocal<>("Current transaction name");
//事务只读状态
private static final ThreadLocal<Boolean> currentTransactionReadOnly =
new NamedThreadLocal<>("Current transaction read-only status");
//事务隔离级别
private static final ThreadLocal<Integer> currentTransactionIsolationLevel =
new NamedThreadLocal<>("Current transaction isolation level");
//事务活跃状态
private static final ThreadLocal<Boolean> actualTransactionActive =
new NamedThreadLocal<>("Actual transaction active");
//......
}DataSourceTransactionManager.doBegin
1 | protected void doBegin(Object transaction, TransactionDefinition definition) { |
该方法的核心操作是:
1、获取新的数据库连接对象,设置ConnectionHolder
2、在当前线程的ThreadLocal变量resources中设置key-value(key=DataSource对象,value=ConnectionHolder)
2.2、commit提交事务
- AbstractPlatformTransactionManager.commit
1 | public final void commit(TransactionStatus status) throws TransactionException { |
- AbstractPlatformTransactionManager.processCommit
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81private void processCommit(DefaultTransactionStatus status) throws TransactionException {
try {
boolean beforeCompletionInvoked = false;
try {
boolean unexpectedRollback = false;
//3个前置操作
prepareForCommit(status);
triggerBeforeCommit(status);
triggerBeforeCompletion(status);
beforeCompletionInvoked = true;
//有保存点,即嵌套事务
if (status.hasSavepoint()) {
if (status.isDebug()) {
logger.debug("Releasing transaction savepoint");
}
unexpectedRollback = status.isGlobalRollbackOnly();
//释放保存点
status.releaseHeldSavepoint();
}
//新事务
else if (status.isNewTransaction()) {
if (status.isDebug()) {
logger.debug("Initiating transaction commit");
}
unexpectedRollback = status.isGlobalRollbackOnly();
//执行提交
doCommit(status);
}
//全局回滚失败
else if (isFailEarlyOnGlobalRollbackOnly()) {
unexpectedRollback = status.isGlobalRollbackOnly();
}
// Throw UnexpectedRollbackException if we have a global rollback-only
// marker but still didn't get a corresponding exception from commit. if (unexpectedRollback) {
throw new UnexpectedRollbackException(
"Transaction silently rolled back because it has been marked as rollback-only");
}
}
catch (UnexpectedRollbackException ex) {
// can only be caused by doCommit
//触发完成后事务同步,状态为回滚
triggerAfterCompletion(status, TransactionSynchronization.STATUS_ROLLED_BACK);
throw ex;
}
catch (TransactionException ex) {
// can only be caused by doCommit
//提交失败回滚
if (isRollbackOnCommitFailure()) {
doRollbackOnCommitException(status, ex);
}
else {
//触发完成后回调,事务同步状态为未知
triggerAfterCompletion(status, TransactionSynchronization.STATUS_UNKNOWN);
}
throw ex;
}
catch (RuntimeException | Error ex) {
//如果3个前置步骤没有执行完,调用前置最后一步操作
if (!beforeCompletionInvoked) {
triggerBeforeCompletion(status);
}
doRollbackOnCommitException(status, ex);
throw ex;
}
// Trigger afterCommit callbacks, with an exception thrown there
// propagated to callers but the transaction still considered as committed. try {
triggerAfterCommit(status);
}
finally {
triggerAfterCompletion(status, TransactionSynchronization.STATUS_COMMITTED);
}
}
finally {
cleanupAfterCompletion(status);
}
}
2.3、rollback回滚事务
- AbstractPlatformTransactionManager.processRollback
1 | private void processRollback(DefaultTransactionStatus status, boolean unexpected) { |
-------------本文结束,感谢您的阅读-------------
本文作者:
陈进涛
本文链接: https://zhizhi123.com/2021/11/07/spring-transaction-core-implementation/
版权声明: 本作品采用 署名—非商业性使用—相同方式共享 4.0 协议国际版 进行许可。转载请注明出处!
本文链接: https://zhizhi123.com/2021/11/07/spring-transaction-core-implementation/
版权声明: 本作品采用 署名—非商业性使用—相同方式共享 4.0 协议国际版 进行许可。转载请注明出处!
